TypeScript: keyof / typeof
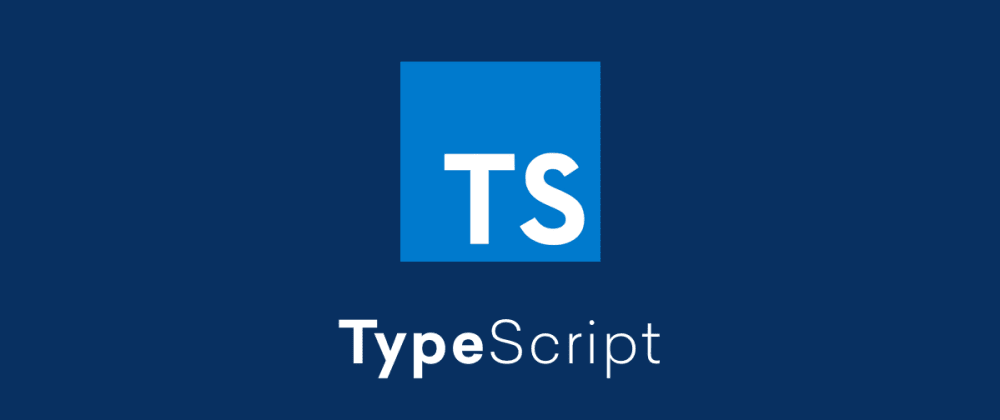
Lets talk about the usage of keyof and typeof in TypeScript. First of all we need to understand the basics of literal types and union of literal types.
Literal types
Basically it allow us to specify exact value that a type can have, it can be one of these 3 types string, number, boolean.
In practice literal types are combines with union types, type guards, and type aliases in order to get an enum-like behavior
keyof
Returns a new type of T that is a union of literal types and these literal types are the names of the properties of T.
With using the keyof operator on the type User, this will return a new union of literal types “id” | “name” | “age” which are the properties of the interface User.
keyof can be used with classes as well. but it works only with public members of the class.
typeof
As you know JavaScript has a typeof operator, but in TypeScript it behave differently.
typeof & keyof
These operators can be used together when we don’t know the type of an object. let see the below example:
Here is another example of typeof keyof on an enum
And here is an example with Generic type. In the following example, we have a function checkData that checks all properties and returns an object with each key and a boolean value.
Thanks for reading — I hope you found this article useful. Happy coding! :)